Bonjour,
J'ai réaliser un programme qui à pour but d'afficher un client, une commande, et une liste de commande en attente. J'ai réaliser une fonction affichage pour les commandes et les clients cependant cet affichage à des bug (numéro apparaissant par " magie" , des tabulation au mauvais endroits) ... Pouvez-vous m'aider ??
De plus losque j'essaye d'ajouter à ma fonction un affichage pour la liste de commande en attente un message d'erreur d'invalid operand sort, cependant je ne voit pas comment la rectifier. Je vous prit de regarder mon code qui suit ainsi que les photos qui accompagne ce post.
Merci d'avance Cordialement.
tp.h
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
typedef struct
{
char idArticle[6];
char denomination[30];
float prixU;
int quantEnStock;
}Article;
typedef struct
{
char idClient[6];
char civilite[4];
char nom[25];
char prenom[25];
int codePostal;
char ville[30];
}Client;
typedef struct
{
char idCommande[5];
char idArticle[5];
char idClient[5];
int quantNonLiv;
}Commande;
typedef struct maillon
{
Commande com;
struct maillon * suiv;
} Maillon,* Liste;
Article lireArt(FILE *fe);
Client lireClient(FILE *fe);
int chargementArt(FILE *fe,Article *tArt[], int max);
Client *chargementCli(FILE *fe,int *tmax, int *nb);
void afficherArt(Article a);
void afficherTArt(Article *tArt[],int max);
void afficherCli(Client c);
void afficheTabCli(Client tabC[], int tmax);
Liste listeVide(void);
Liste ajouterEnTete(Liste l,Commande c);
Liste ajouter(Liste l,Commande c);
void afficheCommande(Commande c);
void afficher(Liste l);
#include "tp.h"
Article lireArt(FILE *fe)
{
Article a;
fgets(a.idArticle,7,fe);
a.idArticle[strlen(a.idArticle)-1]='\0';
fgets(a.denomination,30,fe);
a.denomination[strlen(a.denomination)-1]='\0';
fscanf(fe,"%f%*c%d%*c",&a.prixU,&a.quantEnStock);
return a;
}
Client lireClient(FILE *fe)
{
Client c;
fgets(c.idClient,7,fe);
c.idClient[strlen(c.idClient)-1]='\0';
fscanf(fe,"%s%*c",c.civilite);
fgets(c.nom,25,fe);
c.nom[strlen(c.nom)-1]='\0';
fgets(c.prenom,25,fe);
c.prenom[strlen(c.prenom)-1]='\0';
fscanf(fe,"%d%*c",&c.codePostal);
fgets(c.ville,30,fe);
c.ville[strlen(c.ville)-1]='\0';
return c;
}
int chargementArt(FILE *fe,Article *tArt[], int max)
{
Article a;
int i=0;
a=lireArt(fe);
while(!feof(fe))
{
if(i==max)
{
printf("tableau plein\n");
return -2;
}
tArt=(Article*) malloc (sizeof(Article));
if(tArt[i]==NULL)
{ printf("Problème de malloc \n");
return -1;
}
*tArt[i]=a;
i=i+1;
a=lireArt(fe);
}
return i;
}
Client *chargementCli(FILE *fe,int *tmax, int *nb)
{
int i=0;
Client *tabC,*aux;
*tmax=5;
Client c;
tabC=(Client*)malloc(*tmax*sizeof(Client));
if(tabC==NULL)
{
printf("Pb malloc\n");
return NULL;
}
c=lireClient(fe);
while(!feof(fe))
{
if(i==*tmax)
{
*tmax=*tmax+5;
aux=(Client*)realloc(tabC,*tmax*sizeof(Client));
if(aux==NULL)
{
printf("Pb realloc\n");
return NULL;
}
tabC=aux;
}
tabC[i]=c;
i=i+1;
c=lireClient(fe);
}
*nb=i;
return tabC;
}
void afficherArt(Article a)
{
printf("%s\t%s\t%.2f\t%d\n", a.idArticle,a.denomination,a.prixU,a.quantEnStock);
}
void afficherTArt(Article *tArt[],int max)
{
int i;
for(i=0;i<max;i++)
afficherArt(*tArt[i]);
}
void afficherCli(Client c)
{
printf("%s\t%s\t%s\t%s\t%d\t%s\n",c.idClient,c.civilite,c.nom,c.prenom,c.ville,c.ville);
}
void afficheTabCli(Client tabC[], int tmax)
{
int i;
for(i=0;i<tmax;i++)
afficherCli(tabC[i]);
}
Liste listeVide(void)
{
return NULL;
}
Liste ajouterEnTete(Liste l,Commande c)
{
Maillon *m;
m=(Maillon*)malloc(sizeof(Maillon));
if(m==NULL)
{
printf("problème malloc\n");
exit (1);
}
m->com=c;
m->suiv=l;
return m;
}
Liste ajouter(Liste l,Commande c)
{
if(l==NULL)
return ajouterEnTete(l,c);
if(c==l->com)
return l;
l->suiv=ajouter(l->suiv,c);
return l;
}
void afficheCommande(Commande c)
{
printf("%s\t%s\t%s\t%d\n",c.idCommande,c.idArticle,c.idClient,c.quantNonLiv);
}
void afficher(Liste l)
{ Commande c;
if(listeVide)
{ printf("opération impossible !\n");
return;
}
afficheCommande(c);
afficher(l);
}
testtp.c
#include "tp.h"
void test(void)
{
FILE *fe;
Article *tArt[200], a;
Client *tabC;
Commande c;
int nbArt, tmax, nb;
char nomfich[20];
Liste l;
printf("donnez le nom du fichier : ");
scanf("%s",nomfich);
fe=fopen(nomfich,"r");
if(fe==NULL)
{
printf("Probleme d'ouverture du fichier en lecture\n");
return;
}
if(strcmp(nomfich,"articles.txt")==0)
{ nbArt=chargementArt(fe,tArt, 200);
afficherTArt(tArt,nbArt);
}
if(strcmp(nomfich,"clients.txt")==0)
{ tabC=chargementCli(fe,&tmax,&nb);
afficheTabCli(tabC, nb);
}
if(strcmp(nomfich,"lignesCommandesEncours.txt")==0)
{ l=ajouter(l,c);
afficher(l);
}
fclose(fe);
}
int main(){
test();
return 0;
}
[/i][/i][/i][/i][/i]
J'ai réaliser un programme qui à pour but d'afficher un client, une commande, et une liste de commande en attente. J'ai réaliser une fonction affichage pour les commandes et les clients cependant cet affichage à des bug (numéro apparaissant par " magie" , des tabulation au mauvais endroits) ... Pouvez-vous m'aider ??
De plus losque j'essaye d'ajouter à ma fonction un affichage pour la liste de commande en attente un message d'erreur d'invalid operand sort, cependant je ne voit pas comment la rectifier. Je vous prit de regarder mon code qui suit ainsi que les photos qui accompagne ce post.
Merci d'avance Cordialement.
tp.h
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
typedef struct
{
char idArticle[6];
char denomination[30];
float prixU;
int quantEnStock;
}Article;
typedef struct
{
char idClient[6];
char civilite[4];
char nom[25];
char prenom[25];
int codePostal;
char ville[30];
}Client;
typedef struct
{
char idCommande[5];
char idArticle[5];
char idClient[5];
int quantNonLiv;
}Commande;
typedef struct maillon
{
Commande com;
struct maillon * suiv;
} Maillon,* Liste;
Article lireArt(FILE *fe);
Client lireClient(FILE *fe);
int chargementArt(FILE *fe,Article *tArt[], int max);
Client *chargementCli(FILE *fe,int *tmax, int *nb);
void afficherArt(Article a);
void afficherTArt(Article *tArt[],int max);
void afficherCli(Client c);
void afficheTabCli(Client tabC[], int tmax);
Liste listeVide(void);
Liste ajouterEnTete(Liste l,Commande c);
Liste ajouter(Liste l,Commande c);
void afficheCommande(Commande c);
void afficher(Liste l);
#include "tp.h"
Article lireArt(FILE *fe)
{
Article a;
fgets(a.idArticle,7,fe);
a.idArticle[strlen(a.idArticle)-1]='\0';
fgets(a.denomination,30,fe);
a.denomination[strlen(a.denomination)-1]='\0';
fscanf(fe,"%f%*c%d%*c",&a.prixU,&a.quantEnStock);
return a;
}
Client lireClient(FILE *fe)
{
Client c;
fgets(c.idClient,7,fe);
c.idClient[strlen(c.idClient)-1]='\0';
fscanf(fe,"%s%*c",c.civilite);
fgets(c.nom,25,fe);
c.nom[strlen(c.nom)-1]='\0';
fgets(c.prenom,25,fe);
c.prenom[strlen(c.prenom)-1]='\0';
fscanf(fe,"%d%*c",&c.codePostal);
fgets(c.ville,30,fe);
c.ville[strlen(c.ville)-1]='\0';
return c;
}
int chargementArt(FILE *fe,Article *tArt[], int max)
{
Article a;
int i=0;
a=lireArt(fe);
while(!feof(fe))
{
if(i==max)
{
printf("tableau plein\n");
return -2;
}
tArt=(Article*) malloc (sizeof(Article));
if(tArt[i]==NULL)
{ printf("Problème de malloc \n");
return -1;
}
*tArt[i]=a;
i=i+1;
a=lireArt(fe);
}
return i;
}
Client *chargementCli(FILE *fe,int *tmax, int *nb)
{
int i=0;
Client *tabC,*aux;
*tmax=5;
Client c;
tabC=(Client*)malloc(*tmax*sizeof(Client));
if(tabC==NULL)
{
printf("Pb malloc\n");
return NULL;
}
c=lireClient(fe);
while(!feof(fe))
{
if(i==*tmax)
{
*tmax=*tmax+5;
aux=(Client*)realloc(tabC,*tmax*sizeof(Client));
if(aux==NULL)
{
printf("Pb realloc\n");
return NULL;
}
tabC=aux;
}
tabC[i]=c;
i=i+1;
c=lireClient(fe);
}
*nb=i;
return tabC;
}
void afficherArt(Article a)
{
printf("%s\t%s\t%.2f\t%d\n", a.idArticle,a.denomination,a.prixU,a.quantEnStock);
}
void afficherTArt(Article *tArt[],int max)
{
int i;
for(i=0;i<max;i++)
afficherArt(*tArt[i]);
}
void afficherCli(Client c)
{
printf("%s\t%s\t%s\t%s\t%d\t%s\n",c.idClient,c.civilite,c.nom,c.prenom,c.ville,c.ville);
}
void afficheTabCli(Client tabC[], int tmax)
{
int i;
for(i=0;i<tmax;i++)
afficherCli(tabC[i]);
}
Liste listeVide(void)
{
return NULL;
}
Liste ajouterEnTete(Liste l,Commande c)
{
Maillon *m;
m=(Maillon*)malloc(sizeof(Maillon));
if(m==NULL)
{
printf("problème malloc\n");
exit (1);
}
m->com=c;
m->suiv=l;
return m;
}
Liste ajouter(Liste l,Commande c)
{
if(l==NULL)
return ajouterEnTete(l,c);
if(c==l->com)
return l;
l->suiv=ajouter(l->suiv,c);
return l;
}
void afficheCommande(Commande c)
{
printf("%s\t%s\t%s\t%d\n",c.idCommande,c.idArticle,c.idClient,c.quantNonLiv);
}
void afficher(Liste l)
{ Commande c;
if(listeVide)
{ printf("opération impossible !\n");
return;
}
afficheCommande(c);
afficher(l);
}
testtp.c
#include "tp.h"
void test(void)
{
FILE *fe;
Article *tArt[200], a;
Client *tabC;
Commande c;
int nbArt, tmax, nb;
char nomfich[20];
Liste l;
printf("donnez le nom du fichier : ");
scanf("%s",nomfich);
fe=fopen(nomfich,"r");
if(fe==NULL)
{
printf("Probleme d'ouverture du fichier en lecture\n");
return;
}
if(strcmp(nomfich,"articles.txt")==0)
{ nbArt=chargementArt(fe,tArt, 200);
afficherTArt(tArt,nbArt);
}
if(strcmp(nomfich,"clients.txt")==0)
{ tabC=chargementCli(fe,&tmax,&nb);
afficheTabCli(tabC, nb);
}
if(strcmp(nomfich,"lignesCommandesEncours.txt")==0)
{ l=ajouter(l,c);
afficher(l);
}
fclose(fe);
}
int main(){
test();
return 0;
}
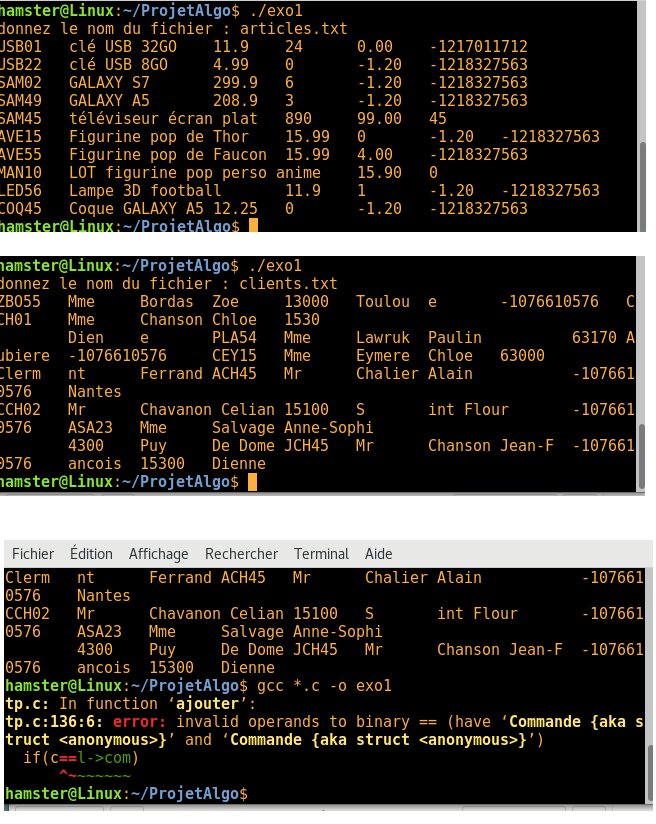