Bonjour,
Mon binôme et moi sommes sur la dernière ligne droite de notre formation et nous travaillons sur un mini projet d'application de jeu de Quizz avec pour chaque Quizz, 10 Questions, pour chaque Question, on peut avoir 4 propositions de réponses.
Notre MCD est bon, nos entités aussi ainsi que leur relations.
Nous avançons en nous répartissant les tâches.
Là j'en suis donc rendu au moment où un utilisateur identifié (donc en Bdd) peut proposer son nouveau Quizz avec toutes les Questions et propositions de réponses.
Voici le Quizz Entity :
Voici le Question Entity :
Au niveau de nos Wireframes, nous souhaitons avoir sur la même page un formulaire qui fait appel à l'entité Quizz et l'entité Question. Donc ce qui fait aussi alors logiquement 2 FormType.
Voici un exemple du rendu de ce que l'on souhaite :
J'ai testé donc avec un QuizzType et un QuestionType
Et du coup dans mon Controller j'ai ceci :
Lorsque je fait un dump($request), je ne récupère que les données de Question:
D'autre part, l'autre problème qui se pose est comment répéter le formulaire Question 10 fois avec les propositions.
Concernant la vue j'ai mis les _formQuizz.html.twig et _formQuestion.html.twig à part et je les ai include dans le template new.html.twig.
On est bien sur des formulaires imbriqués mais comment faire ? Je suis un peu perdue car ce sont des choses qu'on n'a pas forcément vu en cours. Et j'ai beau chercher une solution, je ne trouve pas. C'est pour cette raison que mon dernier recours est le forum.
Si quelqu'un a une piste, une idée, un conseil, ça serait super !
Merci
Modifié par Virginia (15 Sep 2018 - 14:03)
Mon binôme et moi sommes sur la dernière ligne droite de notre formation et nous travaillons sur un mini projet d'application de jeu de Quizz avec pour chaque Quizz, 10 Questions, pour chaque Question, on peut avoir 4 propositions de réponses.
Notre MCD est bon, nos entités aussi ainsi que leur relations.
Nous avançons en nous répartissant les tâches.
Là j'en suis donc rendu au moment où un utilisateur identifié (donc en Bdd) peut proposer son nouveau Quizz avec toutes les Questions et propositions de réponses.
Voici le Quizz Entity :
<?php
namespace App\Entity;
use Doctrine\ORM\Mapping as ORM;
use Doctrine\ORM\Mapping\JoinColumn;
use Doctrine\Common\Collections\Collection;
use Doctrine\Common\Collections\ArrayCollection;
/**
* @ORM\Entity(repositoryClass="App\Repository\QuizzRepository")
*/
class Quizz
{
/**
* @ORM\Id()
* @ORM\GeneratedValue()
* @ORM\Column(type="integer")
*/
private $id;
/**
* @ORM\Column(type="string", length=128)
*/
private $title;
/**
* @ORM\Column(type="string", length=128)
*/
private $slug;
/**
* @ORM\Column(type="text", nullable=true)
*/
private $description;
/**
* @ORM\Column(type="boolean", nullable=true)
*/
private $IsPrivate;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\Category", inversedBy="quizzs")
*/
private $category;
/**
* @ORM\OneToMany(targetEntity="App\Entity\Question", mappedBy="quizz", orphanRemoval=true)
*/
private $questions;
/**
* @ORM\OneToMany(targetEntity="App\Entity\IsLike", mappedBy="quizz")
*/
private $isLikes;
/**
* @ORM\OneToMany(targetEntity="App\Entity\Statistic", mappedBy="quizz", orphanRemoval=true)
*/
private $statistics;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\User", inversedBy="quizzs")
* @ORM\JoinColumn(nullable=false)
*/
private $author;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\Crew", inversedBy="quizzs")
*/
private $crew;
public function __construct()
{
$this->questions = new ArrayCollection();
$this->isLikes = new ArrayCollection();
$this->statistics = new ArrayCollection();
}
public function getId(): ?int
{
return $this->id;
}
public function getTitle(): ?string
{
return $this->title;
}
public function setTitle(string $title): self
{
$this->title = $title;
return $this;
}
public function getSlug(): ?string
{
return $this->slug;
}
public function setSlug(string $slug): self
{
$this->slug = $slug;
return $this;
}
public function getDescription(): ?string
{
return $this->description;
}
public function setDescription(?string $description): self
{
$this->description = $description;
return $this;
}
public function getIsPrivate() : ? bool
{
return $this->IsPrivate;
}
public function setIsPrivate(? bool $IsPrivate) : self
{
$this->IsPrivate = $IsPrivate;
return $this;
}
public function getCategory(): ?Category
{
return $this->category;
}
public function setCategory(?Category $category): self
{
$this->category = $category;
return $this;
}
/**
* @return Collection|Question[]
*/
public function getQuestions(): Collection
{
return $this->questions;
}
public function addQuestion(Question $question): self
{
if (!$this->questions->contains($question)) {
$this->questions[] = $question;
$question->setQuizz($this);
}
return $this;
}
public function removeQuestion(Question $question): self
{
if ($this->questions->contains($question)) {
$this->questions->removeElement($question);
// set the owning side to null (unless already changed)
if ($question->getQuizz() === $this) {
$question->setQuizz(null);
}
}
return $this;
}
/**
* @return Collection|IsLike[]
*/
public function getIsLikes(): Collection
{
return $this->isLikes;
}
public function addIsLike(IsLike $isLike): self
{
if (!$this->isLikes->contains($isLike)) {
$this->isLikes[] = $isLike;
$isLike->setQuizz($this);
}
return $this;
}
public function removeIsLike(IsLike $isLike): self
{
if ($this->isLikes->contains($isLike)) {
$this->isLikes->removeElement($isLike);
// set the owning side to null (unless already changed)
if ($isLike->getQuizz() === $this) {
$isLike->setQuizz(null);
}
}
return $this;
}
/**
* @return Collection|Statistic[]
*/
public function getStatistics(): Collection
{
return $this->statistics;
}
public function addStatistic(Statistic $statistic): self
{
if (!$this->statistics->contains($statistic)) {
$this->statistics[] = $statistic;
$statistic->setQuizz($this);
}
return $this;
}
public function removeStatistic(Statistic $statistic): self
{
if ($this->statistics->contains($statistic)) {
$this->statistics->removeElement($statistic);
// set the owning side to null (unless already changed)
if ($statistic->getQuizz() === $this) {
$statistic->setQuizz(null);
}
}
return $this;
}
public function getAuthor(): ?User
{
return $this->author;
}
public function setAuthor(?User $author): self
{
$this->author = $author;
return $this;
}
public function getCrew(): ?Crew
{
return $this->crew;
}
public function setCrew(?Crew $crew): self
{
$this->crew = $crew;
return $this;
}
public function __toString()
{
return $this->title;
}
}
Voici le Question Entity :
<?php
namespace App\Entity;
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity(repositoryClass="App\Repository\QuestionRepository")
*/
class Question
{
/**
* @ORM\Id()
* @ORM\GeneratedValue()
* @ORM\Column(type="integer")
*/
private $id;
/**
* @ORM\Column(type="string", length=255)
*/
private $body;
/**
* @ORM\Column(type="string", length=128)
*/
private $prop1;
/**
* @ORM\Column(type="string", length=128)
*/
private $prop2;
/**
* @ORM\Column(type="string", length=128)
*/
private $prop3;
/**
* @ORM\Column(type="string", length=128)
*/
private $prop4;
/**
* @ORM\Column(type="string", length=255, nullable=true)
*/
private $anecdote;
/**
* @ORM\Column(type="string", length=255, nullable=true)
*/
private $source;
/**
* @ORM\Column(type="boolean")
*/
private $errore;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\Quizz", inversedBy="questions")
* @ORM\JoinColumn(nullable=false)
*/
private $quizz;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\Level", inversedBy="questions")
* @ORM\JoinColumn(nullable=false)
*/
private $level;
public function getId(): ?int
{
return $this->id;
}
public function getBody(): ?string
{
return $this->body;
}
public function setBody(string $body): self
{
$this->body = $body;
return $this;
}
public function getProp1(): ?string
{
return $this->prop1;
}
public function setProp1(string $prop1): self
{
$this->prop1 = $prop1;
return $this;
}
public function getProp2(): ?string
{
return $this->prop2;
}
public function setProp2(string $prop2): self
{
$this->prop2 = $prop2;
return $this;
}
public function getProp3(): ?string
{
return $this->prop3;
}
public function setProp3(string $prop3): self
{
$this->prop3 = $prop3;
return $this;
}
public function getProp4(): ?string
{
return $this->prop4;
}
public function setProp4(string $prop4): self
{
$this->prop4 = $prop4;
return $this;
}
public function getAnecdote(): ?string
{
return $this->anecdote;
}
public function setAnecdote(?string $anecdote): self
{
$this->anecdote = $anecdote;
return $this;
}
public function getSource(): ?string
{
return $this->source;
}
public function setSource(?string $source): self
{
$this->source = $source;
return $this;
}
public function getErrore(): ?bool
{
return $this->errore;
}
public function setErrore(bool $errore): self
{
$this->errore = $errore;
return $this;
}
public function getQuizz(): ?Quizz
{
return $this->quizz;
}
public function setQuizz(?Quizz $quizz): self
{
$this->quizz = $quizz;
return $this;
}
public function getLevel(): ?Level
{
return $this->level;
}
public function setLevel(?Level $level): self
{
$this->level = $level;
return $this;
}
}
Au niveau de nos Wireframes, nous souhaitons avoir sur la même page un formulaire qui fait appel à l'entité Quizz et l'entité Question. Donc ce qui fait aussi alors logiquement 2 FormType.
Voici un exemple du rendu de ce que l'on souhaite :
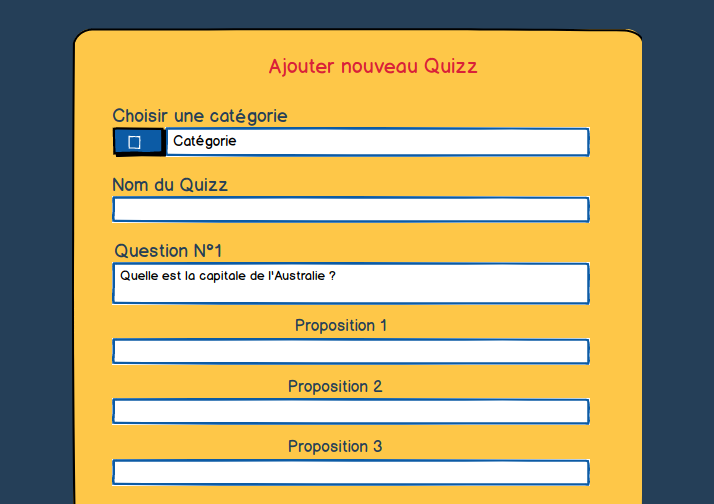
J'ai testé donc avec un QuizzType et un QuestionType
Et du coup dans mon Controller j'ai ceci :
/**
* @Route("/quizz/propose/nouveau", name="quizz_list_new")
*/
public function new(Request $request, ObjectManager $manager)
{
$quizz = new Quizz();
$question = new Question();
$form1 = $this->createForm(QuizzType::class, $quizz);
$form2 = $this->createForm(QuestionType::class, $question);
$form1->handleRequest($request);
$form2->handleRequest($request);
dump($request);
if ($form1->isSubmitted() && $form1->isValid()) {
$manager->persist($quizz);
$manager->persist($question);
$manager->flush();
return $this->redirectToRoute('quizz_list_show');
}
dump($request);
return $this->render('quizz/new.html.twig', [
'form1'=>$form1->createView(),
'form2' => $form2->createView()
]);
}
Lorsque je fait un dump($request), je ne récupère que les données de Question:
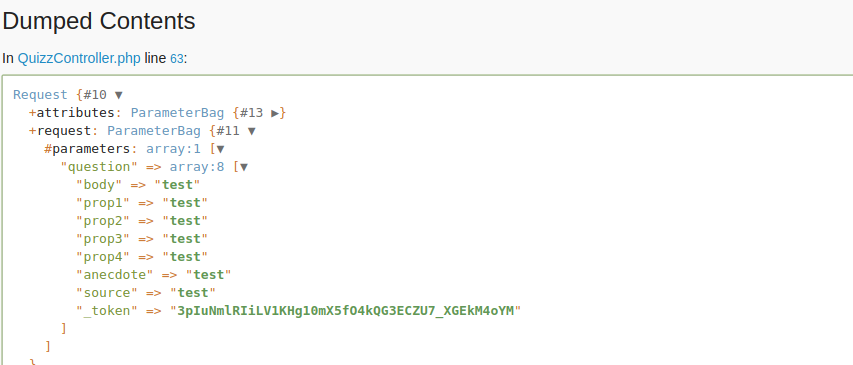
D'autre part, l'autre problème qui se pose est comment répéter le formulaire Question 10 fois avec les propositions.
Concernant la vue j'ai mis les _formQuizz.html.twig et _formQuestion.html.twig à part et je les ai include dans le template new.html.twig.
On est bien sur des formulaires imbriqués mais comment faire ? Je suis un peu perdue car ce sont des choses qu'on n'a pas forcément vu en cours. Et j'ai beau chercher une solution, je ne trouve pas. C'est pour cette raison que mon dernier recours est le forum.
Si quelqu'un a une piste, une idée, un conseil, ça serait super !

Merci
Modifié par Virginia (15 Sep 2018 - 14:03)