Bonjour,
Cela fait 3 semaines que j'apprends Symfony donc tout est encore un peu frais. Donc si quelqu'un pouvait me donner un petit avis, un conseil pour voir où est-ce que je bloque.
Je dois créer ma page d'accueil d'application (dans le genre foires aux questions comme Quora et StackOverflow).
J'ai deux entités Questions et Tags avec une relation ManyToMany :
Entité Tag :
Entité Question :
QuestionController :
Et pour finir ma vue Twig :
Si j'enlève {{questions.tagName}} de ma vue j'ai bien mes questions qui s'affiche avec la date puisque j'ai fait une requete custom dans le repository :
Mais je souhaite aussi afficher les Tags liés aux questions.
Si je laisse {{questions.tagName}} avec une boucle for j'ai une erreur
Auriez-vous une idée de comment je peux sortir de ce problème ? Je pense savoir d'où cela peut venir mais je ne trouve pas comment m'y prendre ?
Merci
Cela fait 3 semaines que j'apprends Symfony donc tout est encore un peu frais. Donc si quelqu'un pouvait me donner un petit avis, un conseil pour voir où est-ce que je bloque.
Je dois créer ma page d'accueil d'application (dans le genre foires aux questions comme Quora et StackOverflow).
J'ai deux entités Questions et Tags avec une relation ManyToMany :
Entité Tag :
<?php
namespace App\Entity;
use Doctrine\Common\Collections\ArrayCollection;
use Doctrine\Common\Collections\Collection;
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity(repositoryClass="App\Repository\TagRepository")
*/
class Tag
{
/**
* @ORM\Id()
* @ORM\GeneratedValue()
* @ORM\Column(type="integer")
*/
private $id;
/**
* @ORM\Column(type="string", length=64)
*/
private $tagName;
/**
* @ORM\ManyToMany(targetEntity="App\Entity\Question", mappedBy="tags")
*/
private $questions;
public function __construct()
{
$this->questions = new ArrayCollection();
}
public function getId(): ?int
{
return $this->id;
}
public function getTagName(): ?string
{
return $this->tagName;
}
public function setTagName(string $tagName): self
{
$this->tagName = $tagName;
return $this;
}
/**
* @return Collection|Question[]
*/
public function getQuestions(): Collection
{
return $this->questions;
}
public function addQuestion(Question $question): self
{
if (!$this->questions->contains($question)) {
$this->questions[] = $question;
$question->addTag($this);
}
return $this;
}
public function removeQuestion(Question $question): self
{
if ($this->questions->contains($question)) {
$this->questions->removeElement($question);
$question->removeTag($this);
}
return $this;
}
}
Entité Question :
<?php
namespace App\Entity;
use Doctrine\Common\Collections\ArrayCollection;
use Doctrine\Common\Collections\Collection;
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity(repositoryClass="App\Repository\QuestionRepository")
*/
class Question
{
/**
* @ORM\Id()
* @ORM\GeneratedValue()
* @ORM\Column(type="integer")
*/
private $id;
/**
* @ORM\Column(type="string", length=255)
*/
private $titleQuestion;
/**
* @ORM\Column(type="text")
*/
private $description;
/**
* @ORM\OneToMany(targetEntity="App\Entity\Response", mappedBy="question", cascade={"persist"})
*/
private $responses;
/**
* @ORM\ManyToMany(targetEntity="App\Entity\Tag", inversedBy="questions")
*/
private $tags;
/**
* @ORM\Column(type="datetime")
*/
private $createdAt;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\User", inversedBy="questions")
*/
private $user;
public function __construct()
{
$this->responses = new ArrayCollection();
$this->tags = new ArrayCollection();
}
public function getId(): ?int
{
return $this->id;
}
public function getTitleQuestion(): ?string
{
return $this->titleQuestion;
}
public function setTitleQuestion(string $titleQuestion): self
{
$this->titleQuestion = $titleQuestion;
return $this;
}
public function getDescription(): ?string
{
return $this->description;
}
public function setDescription(string $description): self
{
$this->description = $description;
return $this;
}
/**
* @return Collection|Response[]
*/
public function getResponses(): Collection
{
return $this->responses;
}
public function addResponse(Response $response): self
{
if (!$this->responses->contains($response)) {
$this->responses[] = $response;
$response->setQuestion($this);
}
return $this;
}
public function removeResponse(Response $response): self
{
if ($this->responses->contains($response)) {
$this->responses->removeElement($response);
// set the owning side to null (unless already changed)
if ($response->getQuestion() === $this) {
$response->setQuestion(null);
}
}
return $this;
}
/**
* @return Collection|Tag[]
*/
public function getTags(): Collection
{
return $this->tags;
}
public function addTag(Tag $tag): self
{
if (!$this->tags->contains($tag)) {
$this->tags[] = $tag;
}
return $this;
}
public function removeTag(Tag $tag): self
{
if ($this->tags->contains($tag)) {
$this->tags->removeElement($tag);
}
return $this;
}
public function getCreatedAt(): ?\DateTimeInterface
{
return $this->createdAt;
}
public function setCreatedAt(\DateTimeInterface $createdAt): self
{
$this->createdAt = $createdAt;
return $this;
}
public function getUser(): ?User
{
return $this->user;
}
public function setUser(?User $user): self
{
$this->user = $user;
return $this;
}
}
QuestionController :
<?php
namespace App\Controller;
use App\Entity\Tag;
use App\Entity\Question;
use App\Entity\Response;
use App\Repository\QuestionRepository;
use Doctrine\ORM\EntityManagerInterface;
use Symfony\Component\Routing\Annotation\Route;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
class QuestionController extends AbstractController
{
/**
* @Route("/", name="home")
*/
public function index(QuestionRepository $questionRepository)
{
$repository = $this->getDoctrine()->getRepository(Tag::class);
$questions = $questionRepository->findAllQuestionsByDate();
return $this->render('question/index.html.twig', [
'questions'=>$questions
]);
}
Et pour finir ma vue Twig :
{% extends 'base.html.twig' %}
{% block title %}La FAQ-O-Clock !{% endblock %}
{% block body %}
<div class="col-lg-12 container">
<div class="col-lg-12 row">
{% for q in questions %}
<div class="card border-primary my-4 mx-3" style="max-width: 20rem;">
{#% for t in questions.tags %#}
<div class="card-header justify-content {{ cycle(['list-even', 'list-odd', 'list-other'], q.id) }}">{{questions.tagName}}</div>
{#% endfor %#}
<div class="card-body">
<h4 class="card-title">{{q.titleQuestion}}</h4>
<p class="card-text">{{q.createdAt|localizeddate('none', 'none', 'fr', null, 'EEEE d MMMM Y')}}
à
{{q.createdAt|date('H:i')}}</p>
<div class="btn-next d-flex justify-content-end">
<button type="button" class="btn next">Lire la suite</button>
</div>
</div>
</div>
{% endfor %}
{% endblock %}
Si j'enlève {{questions.tagName}} de ma vue j'ai bien mes questions qui s'affiche avec la date puisque j'ai fait une requete custom dans le repository :
public function findAllQuestionsByDate()
{
return $this->createQueryBuilder('q')
->innerJoin()
->orderBy('q.createdAt', 'DESC')
->getQuery()
->getResult();
}
Mais je souhaite aussi afficher les Tags liés aux questions.
Si je laisse {{questions.tagName}} avec une boucle for j'ai une erreur
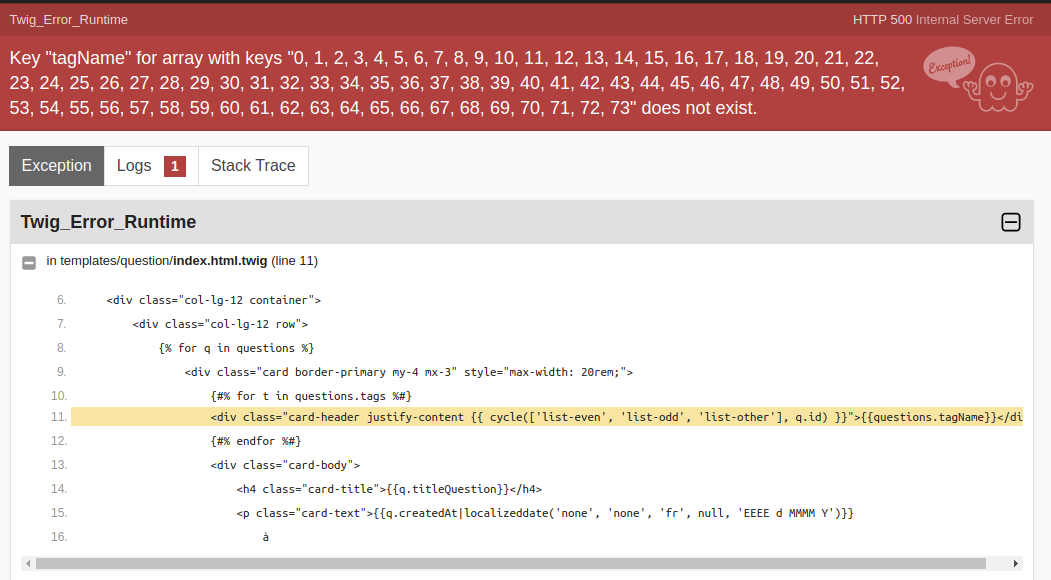
Auriez-vous une idée de comment je peux sortir de ce problème ? Je pense savoir d'où cela peut venir mais je ne trouve pas comment m'y prendre ?
Merci
